Generics in C# allow you to create classes, interfaces, and methods that can work with any data type while maintaining type safety. They provide a way to define classes and methods that defer the specification of one or more types until the class or method is declared and instantiated by client code. This lets you write reusable, type-safe code without sacrificing performance or flexibility.

Here's a basic example of a generic class in C# :
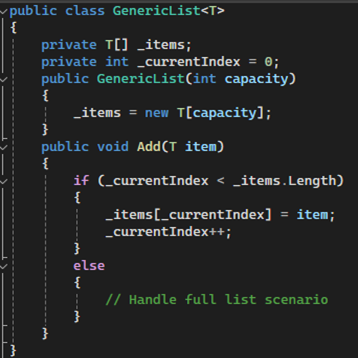
In this example, GenericList<T> is a generic class where T is a placeholder for the type of elements the list will contain. When you create an instance of GenericList, you specify the type of elements it will hold, like so:

This allows you to create lists of integers, strings, or any other type without having to create separate classes for each type.
Generics are widely used in C# for collections (like List<T>, Dictionary<TKey, TValue>, etc.), algorithms, delegates, and many other scenarios where you want to write flexible and type-safe code.
Generic Method :
In C#, a generic method is declared similarly to a regular method but with its own type parameters enclosed in angle brackets before the return type.
These types of parameters can be used as method parameters, return types, local variables, etc.
Generic methods can be part of generic or non-generic classes.
An example of a generic method in C# is Swap<T>(), which swaps the values of two variables of the same type, T.
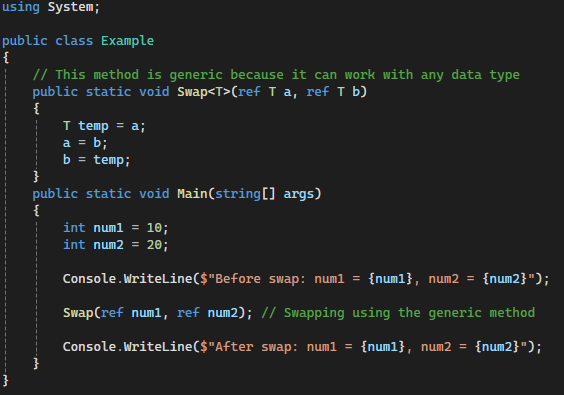
In this example, the Swap method is generic because it can swap variables of any data type. The <T> in the method signature indicates that T is a generic type parameter. When you call this method with int arguments, T becomes int, and the compiler generates code specifically for swapping integers.
Generic Class :
In C#, a generic class is declared using the class keyword followed by one or more type parameters enclosed in angle brackets.
These type parameters can then be used throughout the class definition to specify the types of fields, properties, methods, and constructors.
An example of a generic class in C# is List<T>, where T is the type parameter representing the type of elements stored in the list.
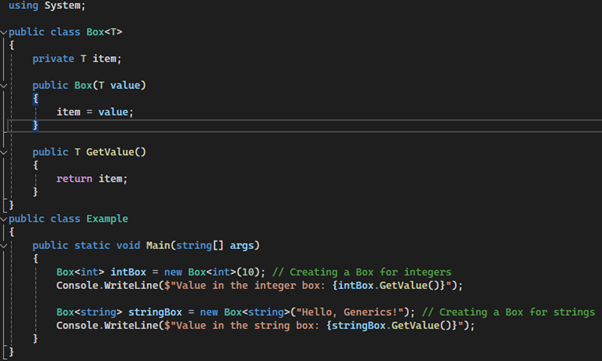
In this example, Box<T> is a generic class. It can hold a value of any data type specified when creating an instance of the class. The <T> in the class declaration indicates that T is a generic type parameter. When you create a Box<int>, T becomes int, and when you create a Box<string>, T becomes string.
留言