Essential Data Structures: Arrays, Lists, and Stacks | Algority Technologies
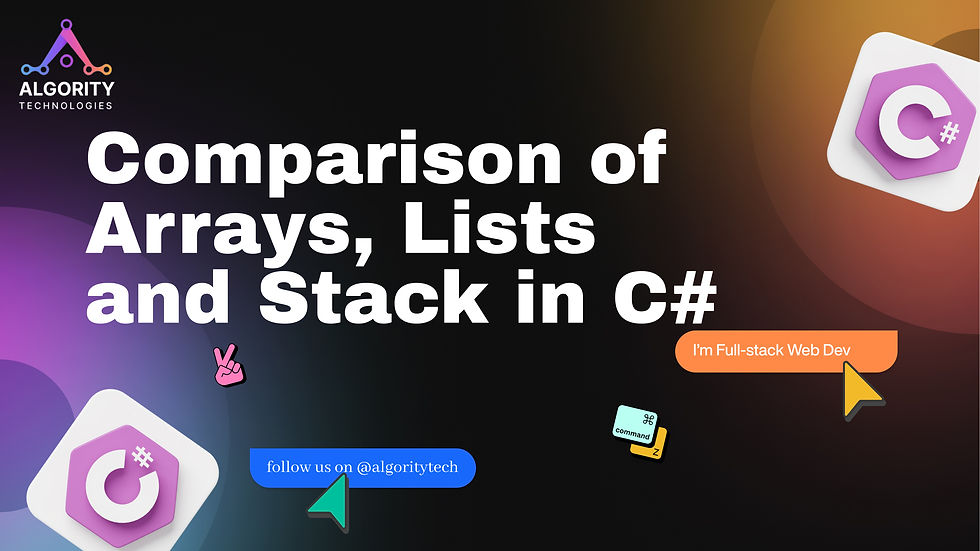
Explore the basics of arrays, lists, and stacks with Algority Technologies! From efficient data storage to dynamic memory allocation, learn the essentials for mastering these fundamental data structures. Perfect for beginners and seasoned developers alike!
Arrays :
An array is a structured data entity of a predetermined size, wherein elements of uniform type are stored consecutively within memory. Each element is accessed by its index. In C#, the array index starts from 0.
Arrays usually have a fixed size set at declaration but some languages provide dynamic or resizable arrays, enabling size changes post declaration.
Arrays offer better performance for random access due to direct memory addressing.
Arrays are suitable for scenarios where the size is known beforehand and direct access to elements is required.
Arrays store elements of a specific data type, ensuring type safety.
Arrays support basic operations like element insertion, deletion, and modification, but resizing can be inefficient.
Namespace:
'Array' is a type, not a namespace. This is why we use namespace using System;
System. Array provides a common base class for all array types in .NET. It defines functionality for creating, manipulating, and accessing arrays.
Example: How to declare an array –
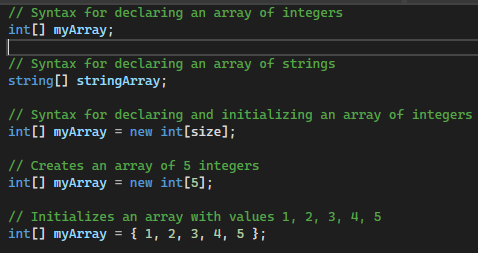
Example of an array in C# :
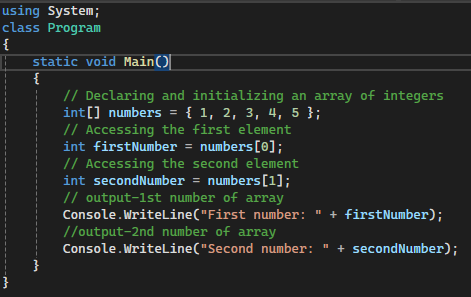
C# Array Properties:
IsFixedSize - It is used to determine whether the array has a fixed size or not.
IsReadOnly - It is used to check whether the Array is read-only or not.
Length - It is used to retrieve the total number of elements across all dimensions of the array.
C# Array Methods:
Sort(Array) - The function Sort(Array) arranges the elements within a one-dimensional Array in ascending order.
Reverse(Array) – It is used to reverse the sequence of elements in a one-dimensional array.
ToString() – This method is employed to retrieve a string representation of the current object.
Example of Sort() :
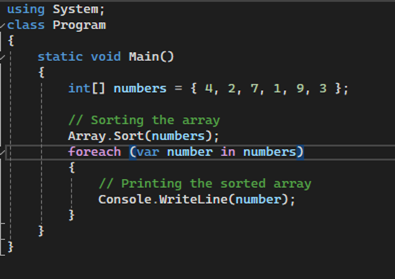
Some more functionalities of an Array
Searching for Elements:
The Array class furnishes techniques for locating elements within an array.
IndexOf retrieves the index of the initial appearance of a designated value.

BinarySearch offers another approach to searching, necessitating prior sorting of the array. Employing a binary search algorithm, it delivers expedited results.
Copying Arrays:
Copying elements from one array to another is achievable via the Copy method:
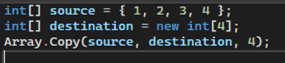
After this operation, the destination will contain the same elements as the source.
Clearing Arrays:
To populate an array with a default value, you can utilize the Clear method.

This will set all elements in numbers to their default value (in this, integers will be set to 0).
Resizing Arrays:
While arrays in C# typically have a fixed size, you can simulate resizing using the Resize method.

This method expands the size of the array to accommodate additional elements.
Checking Equality:
The Array class permits comparing arrays for equality using the Equals method.

Converting Arrays to Lists:
You can convert an array to a List using the ToList method.

Lists :
A list is a dynamic data structure that stores elements of possibly different types sequentially in memory. Each element is connected to its adjacent elements by pointers. In C#, you declare a list using the List<T> class from the System.Collections.Generic namespace, where T represents the type of elements the list will hold.
Lists have the capability to adjust their size dynamically to accommodate additional elements.
Lists support only sequential access.
Lists are generic, allowing storage of different types.
Lists use dynamic memory allocation, potentially non-contiguous.
Lists offer efficient resizing for dynamic operations.
Lists are suitable for scenarios requiring flexibility in size and operations.
Lists are commonly used for collections requiring dynamic manipulation.
Suitable when the data size is not known in advance or when frequent insertions and deletions are expected.
Namespace:
We first import the System.Collections.Generic namespace to use lists.
Example: How to declare a list –
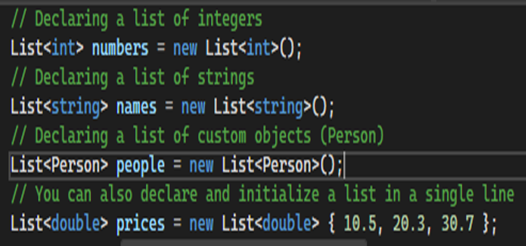
Code Example:
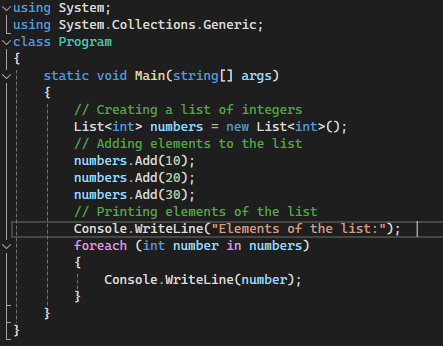
List’s Methods:
Let’s take an example to understand the list’s methods. We’ll create a new list called planets.
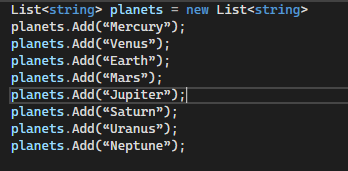
Syntax | Description | Example |
Add() | Adds an item to the List | planets.Add(“pluto”); will add pluto in planet list |
Contains() | Checks to see if the List contains a given item, returning a Boolean | planets.Contains(“Mars”); will return True |
Remove() | It deletes the initial instance of a specified object. | planets.Remove(“pluto”); |
Sort() | Rearrange the elements of a List into ascending order. | planets.Sort(“pluto”); |
Stack:
A stack adheres to the Last-In-First-Out (LIFO) principle, serving as a data structure.Elements can solely be added or removed from the top of the stack.
stacks use dynamic memory allocation, which may not be contiguous and could result in memory fragmentation.
Stacks support operations like push (adding an item to the top) and pop (removing an item from the top) efficiently.
stacks are better suited for dynamic operations like insertion and deletion, especially when the size is unpredictable.
Stacks are used for Last-In-First-Out (LIFO) operations, such as managing method calls or tracking undo operations.
Stacks are generic, allowing storage of different types.
Stacks are often implemented using arrays with dynamic resizing.
Stacks offer efficient push and pop operations.
Stacks are ideal for managing method calls and undo operations.
Namespace:
We first import the System.Collections.Generic namespace to use stack.
Example: How to declare a list –
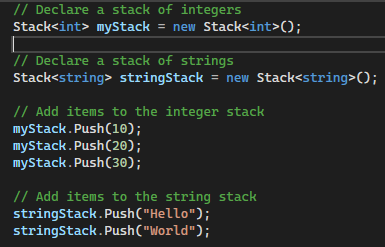
// Accessing elements
// Retrieves the top item without removing it
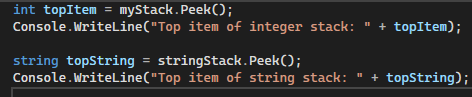
// Remove items from the stack
// Removes and returns the top item

List’s Methods:
Push: It pushes an item onto the top of the stack.

Pop: Extracts and retrieves the element positioned at the top of the stack.

Peek: Returns the item at the top of the stack without removing it.

Count: Obtains the count of elements present within the stack.

Clear: Removes all items from the stack.

Contains: This function assesses whether a specific element exists within the stack.

ToArray: Duplicates the elements of the stack into a fresh array.

TrimExcess: Sets the capacity to the actual number of elements in the stack, if it is less than a threshold value.

Here is the brief differentiation between Arrays, Lists and Stacks.
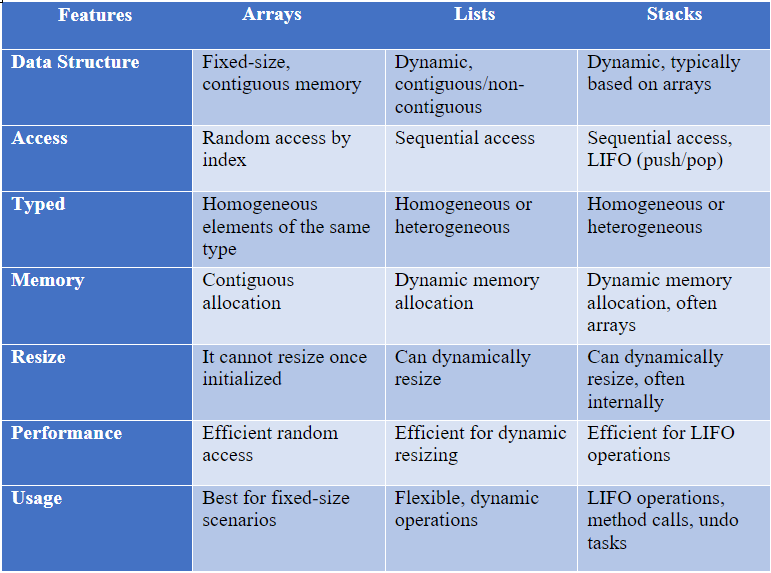
Comments